ESP32 - Dual Temperature Sensor (DS18B20)
How to build Dual temperature Sensor and connect it to Smart-Konnect ?
For the overall instructions, please follow the above video.
The Schema
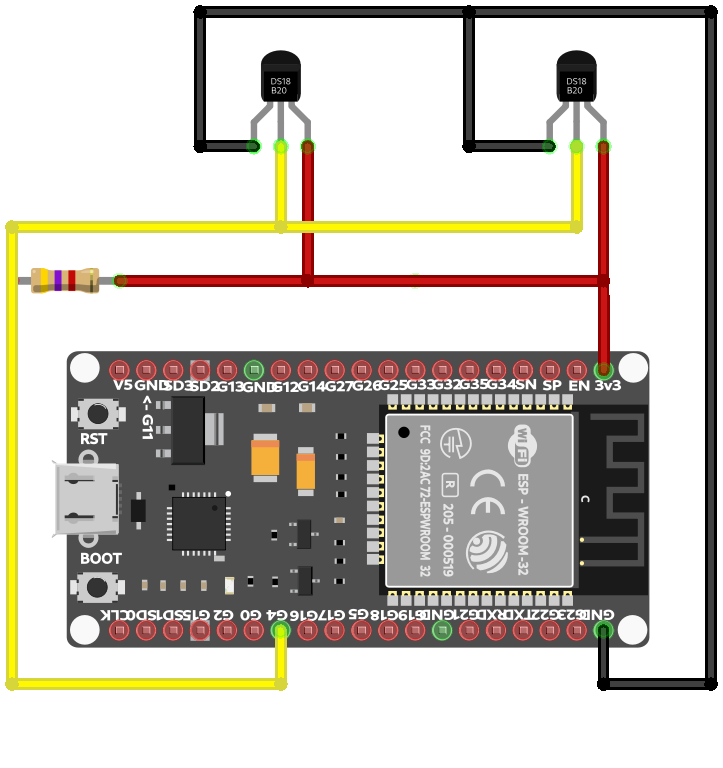
Assembly List
Label | Part Type | Properties |
---|---|---|
DS1 | DS18B20 1-Wire Temperature Sensor | part # DS18B20 |
DS2 | DS18B20 1-Wire Temperature Sensor | part # DS18B20 |
R1 | 4.7kΩ Resistor | package 1206 [SMD]; tolerance ±5%; resistance 4.7kΩ |
U1 | ESP-WROOM-32 | type NodeMCU-32S; variant Generic; pins 38; part # ESP-WROOM-32 |
Shopping List
Amount | Part Type | Properties |
---|---|---|
2 | DS18B20 1-Wire Temperature Sensor | part # DS18B20 |
1 | 4.7kΩ Resistor | package 1206 [SMD]; tolerance ±5%; resistance 4.7kΩ |
1 | ESP-WROOM-32 | type NodeMCU-32S; variant Generic; pins 38; part # ESP-WROOM-32 |
The Code
//################# LIBRARIES ################
/*Libraries*/
#include <Wire.h>
#define ETH_CLK_MODE ETH_CLOCK_GPIO17_OUT
#define ETH_PHY_POWER 12
#include <WiFi.h>
#include <HTTPClient.h>
#include <OneWire.h>
#include <DallasTemperature.h>
/*Constant Variables*/
#define Attempts 10 /* Nº of conection retries */
#define uS_TO_S_FACTOR 1000000 /* Conversion factor for micro seconds to seconds */
#define TIME_TO_SLEEP 60/* Time ESP32 will go to sleep (in seconds) */
/*Sensorial Data to be uploaded*/
float temp1;
float temp2;
/*Smart Konnect - URL & User credentials*/
String iotserver="<YOUR SMART-KONNECT URL GOES HERE>"; // Plataform URL
String my_api_id = "<YOUR API-ID GOES HERE>"; // Insert your ID here
String my_api_key = "<YOUR API-KEY GOES HERE>"; //Insert your API_KEY here
/* WIFI Information */
const char* ssid = "<YOUR WIFI SSID GOES HERE>"; //Insert your Wifi SSID here
const char* pass ="<YOUR WIFI PASSWORD GOES HERE>"; //Insert Wifi password here
void SK_upload_ESP(){
int httpCode;
String payload;
/*Build Header & Begin Connection*/
HTTPClient http; //Declare object of class HTTPClient
http.begin(iotserver); //Specify request destination
http.addHeader("Content-Type", "application/json");
/*Build paylod*/
String sendInfo="{\"api_id\": \""+String(my_api_id)+"\",";
sendInfo+="\"api_key\": \""+String(my_api_key)+"\",";
sendInfo+="\"temperature1\": \""+String(temp1)+"\",";
sendInfo+="\"temperature2\": \""+String(temp2)+"\"}";
Serial.println(sendInfo);
/*Post payload*/
httpCode = http.POST(sendInfo); //Send the request
payload = http.getString(); //Get the response payload
/*Print Result*/
Serial.println(httpCode); //Print HTTP return code
Serial.println(payload); //Print request response payload
/*Close Connection*/
http.end();
}
void StartWiFi(const char* ssid, const char* password)
{
int connAttempts = 0;
Serial.print(F("\r\nConnecting to: ")); Serial.println(String(ssid));
WiFi.begin(ssid, password);
while ((WiFi.status() != WL_CONNECTED) and (connAttempts<Attempts))
{
delay(500);
Serial.print(".");
connAttempts++;
}
if (WiFi.status() != WL_CONNECTED) {
Serial.println(F("Connection Failed!"));
}
else{
Serial.print(F("WiFi connected with IP: "));
Serial.println(WiFi.localIP());
Serial.println(WiFi.RSSI());
}
}
// Data wire is plugged TO GPIO 4
#define ONE_WIRE_BUS 4
// Setup a oneWire instance to communicate with any OneWire devices (not just Maxim/Dallas temperature ICs)
OneWire oneWire(ONE_WIRE_BUS);
// Pass our oneWire reference to Dallas Temperature.
DallasTemperature sensors(&oneWire);
void setup(){
// start serial port
Serial.begin(115200);
// Start up the library
sensors.begin();
}
void loop(){
while (true){
if (WiFi.status() == WL_CONNECTED){
sensors.requestTemperatures();
temp1 = sensors.getTempFByIndex(0);
temp2 = sensors.getTempFByIndex(1);
Serial.println(temp1);
Serial.println(temp2);
SK_upload_ESP();
delay(60000);
}else{
/*Connect Wifi*/
StartWiFi(ssid, pass);
delay(500);
}
}
}